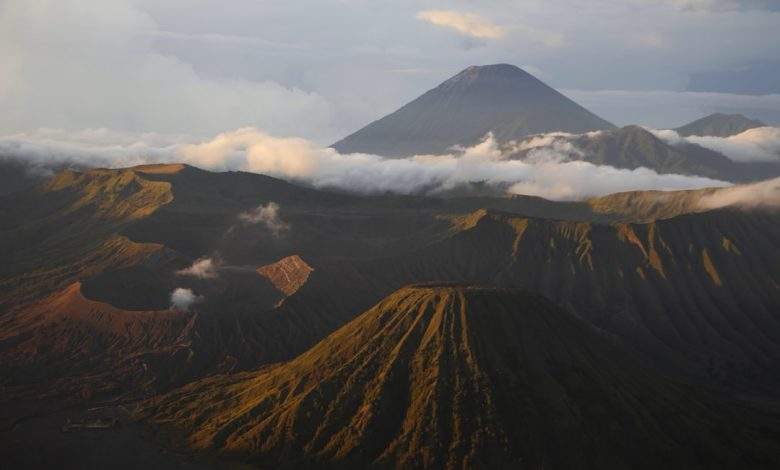
What is the difference between authentication and authorization in Java?
“`html
In the world of Java security, two fundamental concepts stand out: authentication and authorization. While often used interchangeably, these terms serve distinct roles in securing applications. Authentication ensures that an entity is genuinely who or what it claims to be, whereas authorization determines what resources and operations that entity is permitted to access. Understanding the differences between these two security measures is essential for developers building secure Java applications.
Authentication: Verifying the Identity
Authentication is the process of confirming the identity of a user or system attempting to access an application. It answers the question: “Who are you?” This verification process is commonly implemented through various methods, including:
- Username and password: The most commonly used authentication method.
- Multi-factor authentication (MFA): Requires additional verification steps, such as a code sent to a mobile phone.
- Biometric authentication: Uses fingerprints or facial recognition to validate identity.
- OAuth and OpenID Connect: Enables authentication through third-party services.
In Java applications, authentication is often managed using frameworks such as Spring Security and Java Authentication and Authorization Service (JAAS). These libraries provide mechanisms for user login, session management, and integration with external identity providers.
Authorization: Controlling Access
Once a user or system has been authenticated, the next step is authorization. Authorization determines what resources a given user is permitted to access and what actions they can perform. It answers the question: “What are you allowed to do?”
Java applications typically implement authorization using role-based access control (RBAC) or attribute-based access control (ABAC). These models ensure that users have the appropriate privileges based on their roles or attributes.
- RBAC (Role-Based Access Control): Assigns permissions based on predefined roles. For example, an “admin” role may have full access, while a “user” role has limited privileges.
- ABAC (Attribute-Based Access Control): Grants access based on user attributes such as department, job title, or security clearance level.
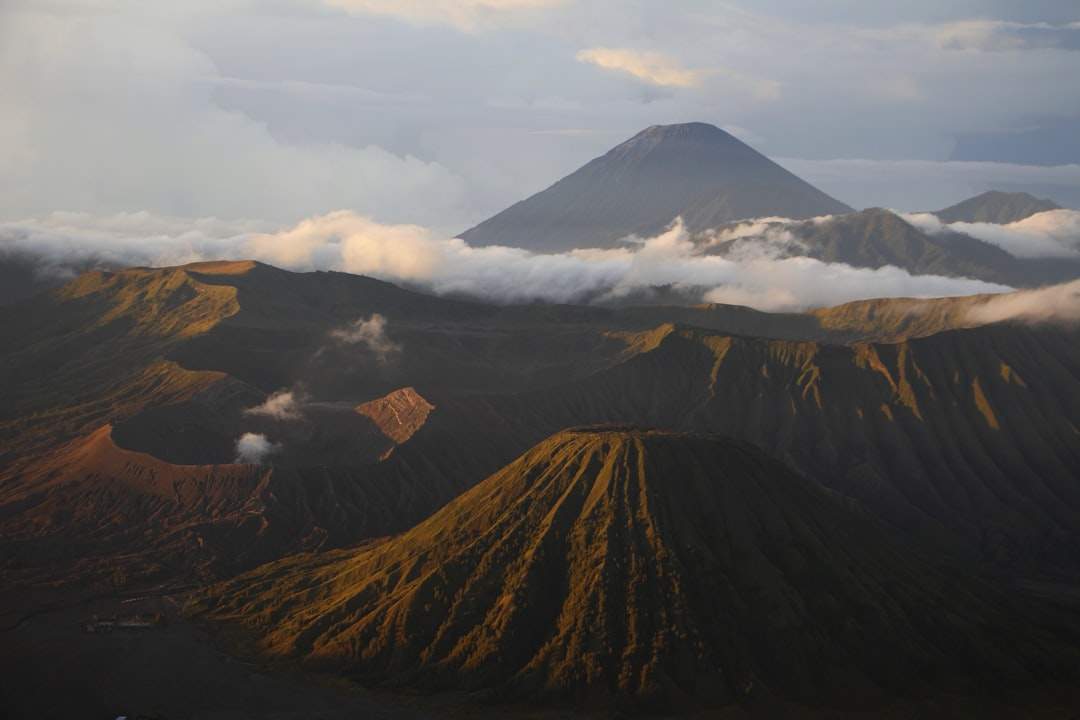
Key Differences Between Authentication and Authorization
The table below highlights the core differences between these two security concepts:
Feature | Authentication | Authorization |
---|---|---|
Purpose | Verifies identity | Determines access rights |
Question Answered | “Who are you?” | “What can you do?” |
Implementation | Login credentials, biometrics, OAuth | Permissions, roles, access policies |
When It Occurs | Before access to the system | After authentication |
In many Java applications, authentication is handled through dedicated authentication providers, while authorization logic is enforced at the controller or service level.
How Authentication and Authorization Work Together
Authentication and authorization work hand in hand to secure applications. Without authentication, an unauthorized user could attempt to access the system. Without authorization, even authenticated users could access sensitive resources they are not supposed to. A well-designed security framework ensures that authentication is performed first, followed by strict authorization checks.
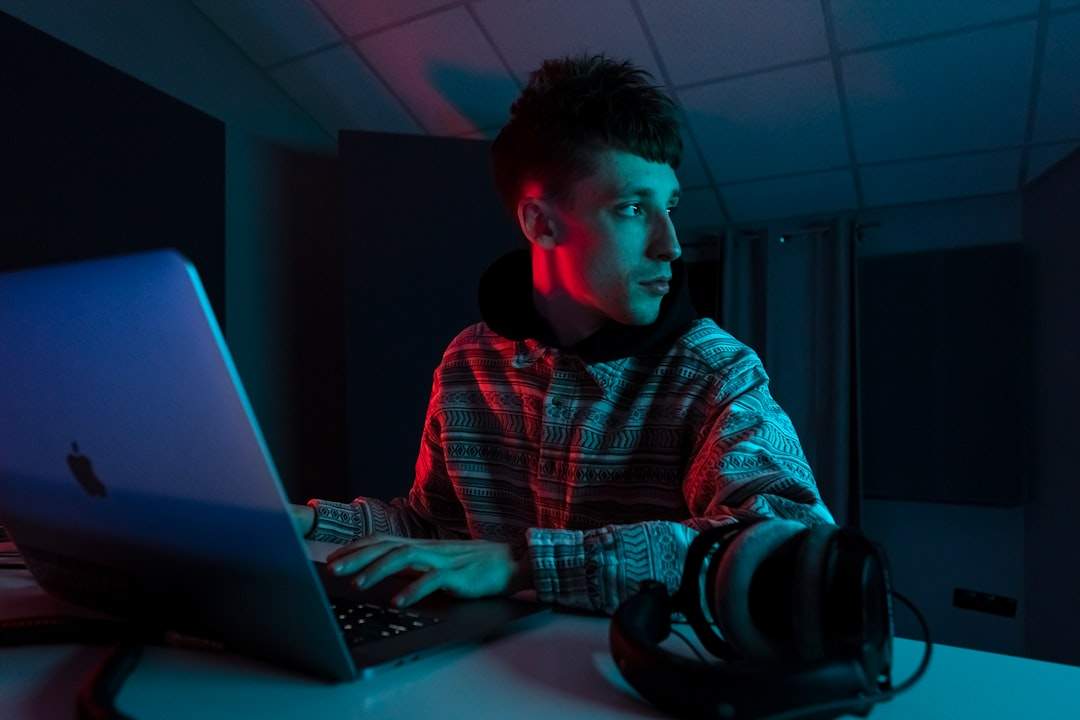
Implementing Security in Java
Java provides several tools and frameworks for implementing authentication and authorization:
- Spring Security: A powerful framework for managing authentication and authorization in Java applications.
- JAAS (Java Authentication and Authorization Service): A built-in API for handling security in Java applications.
- Keycloak: A popular identity provider offering authentication and role-based access control.
Using these tools, developers can implement secure login mechanisms, enforce access rules, and protect sensitive resources from unauthorized access.
FAQ
If authentication succeeds but authorization fails, the user is identified but does not have the necessary permissions to access the requested resources. Typically, an HTTP 403 Forbidden response is returned in web applications.
Yes, a system can implement authentication without authorization. In such a case, users are simply verified but not restricted from performing any actions. This is common in open-access systems where authentication is required for tracking purposes rather than access control.
Spring Security is widely regarded as the best framework for managing both authentication and authorization in Java applications due to its flexibility and extensive features.
OAuth is primarily an authorization framework, though it is often used in conjunction with OpenID Connect for authentication.
“`